4.2.2.5 Establish a Database Connection
Now we have a clear picture and understanding of the fundamentals in the DriverManager and Driver classes as well as the related database connection methods. As we discussed in the previ-ous sections, to connect to a database, two methods, DriverManager.getConnection() and Driver.connect(), can be used. However, as we know, the first method is better than the second one; therefore, in this section, we will concentrate on the use of the first method to establish a database connection.
Figure 4.4 shows a piece of example code to establish a connection using the DriverManager. getConnection() method. This code is a follow-up to the code shown in Figure 4.3—in other words, a valid driver has been loaded and registered before the following connection can be established.
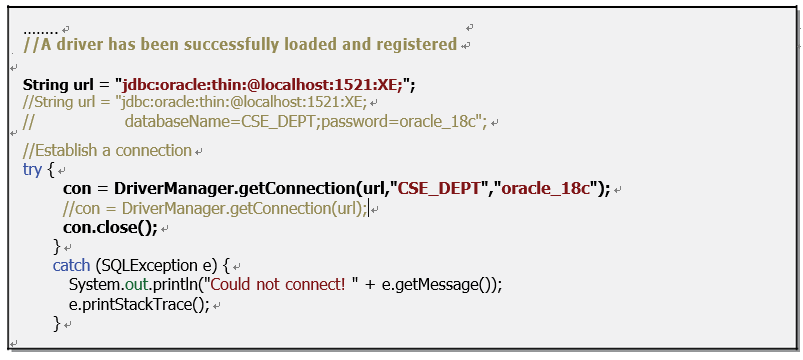
FIGURE 4.4 Example code for the database connection.
Since the DriverManager.getConnection() method is an overloaded method with three different signatures, here we used two of them; the first one is highlighted in bold, and the second one is commented out.
To establish a database connection, a valid JDBC URL is defined in the first codeline with the following components:
- The protocol name jdbc
- The sub-protocol oracle:thin
- The subname localhost:1521:XE
- The database name CSE _ DEPT
Then a try-catch block is used to try to establish a connection using the getConnec-tion() method with three arguments: URL, username and password. After a valid connection is established, a Connection object is returned, and this returned object has the following functions and properties:
1) The Connection object represents an Oracle session with the database.
2) The Connection object provides methods for the creation of Statement objects that will be used to execute Oracle statements in the next step.
3) The Connection object also contains methods for the management of the session, such as transaction locking, catalog selection and error handling.
By definition, the responsibility of a Connection object is to establish a valid database con-nection with your Java application, and that is all. The Connection object has nothing to do with Oracle statement execution. Oracle statement execution is the responsibility of the Statement, PreparedStatement and CallableStatement objects. As we mentioned, both PreparedStatement and CallableStatement are subclasses of the Statement class, and they play different roles in statement execution.
In the next codeline in Figure 4.4, a close() method that belongs to the Connection class is called to try to close a connection. In fact, it is unnecessary to close a connected database in actual applications. However, we use this method here to show users a complete picture of using the Connection object, which means that you must close a connection when it is no longer to be used in
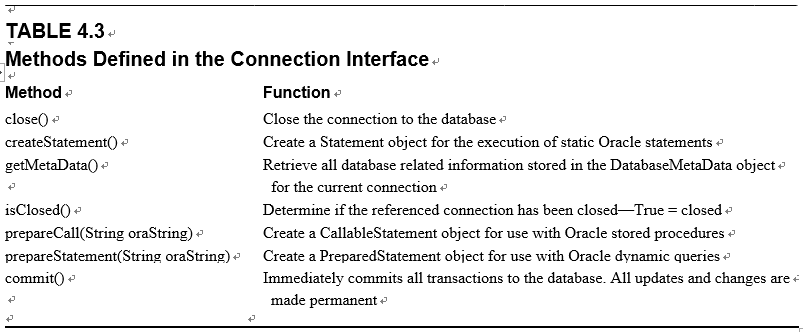
your application (even in the connection pooling situation, but it will not really be closed; instead, it is placed into a pool); otherwise, a runtime error may be encountered when you reuse this connec-tion in the future. Therefore, this codeline is only for testing purposes and should be removed in a real application.
The catch block is used to detect any possible exceptions and display them if any occurred.
A Connection class contains 19 methods, and Table 4.3 lists the 7 most popular methods.
Now a valid database connection has been established, and the next step is to execute Oracle statements to perform data actions against our connected database.